一, 文件上传处理
1,处理方式
- (1)找到文件对应的 input 标签。
- (2)定位到对应的文件地址,使用`send_keys方法进行文件上传。
2,代码示例
package action;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.function.Executable;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import java.time.Duration;
import java.util.ArrayList;
import java.util.List;
import static java.lang.Thread.sleep;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.equalTo;
public class FileDownTest {
static WebDriver driver;
List<Executable> executableList = new ArrayList<>();
@BeforeAll
static void setupClass() {
ChromeOptions options = new ChromeOptions();
options.addArguments("--remote-allow-origins=*");
//创建一个driver对象
driver = new ChromeDriver(options);
//声明隐式等待
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(6));
}
@AfterAll
static void tearDownClass() throws InterruptedException {
sleep(5000);
driver.quit();
}
@Test
void testFileDown() throws InterruptedException {
driver.get("https://vip.ceshiren.com/#/ui_study/file_down");
//定位选择文件按钮
sleep(3000);
WebElement fileInput = driver.findElement(By.id("fileInput"));
//上传文件
fileInput.sendKeys("F:\\JavaTest\\接口自动化\\seleniumTest\\Nginx-Dockerfile.txt");
sleep(5000);
//判断文件是否上传成功
WebElement text = driver.findElement(By.xpath("//*[@id='fileInput']/../h1"));
String textText = text.getText();
System.out.println("文件上传成功后的文本信息:" + textText);
assertThat(textText,equalTo("Nginx-Dockerfile.txt"));
}
}
二, Alert 弹框处理
1,处理方式
- (1)如果弹窗可以找到对应元素,直接处理即可。
- (2)如果弹窗无法定位,则需要使用 Alert 对应方法处理。
2,Alert 常用方法
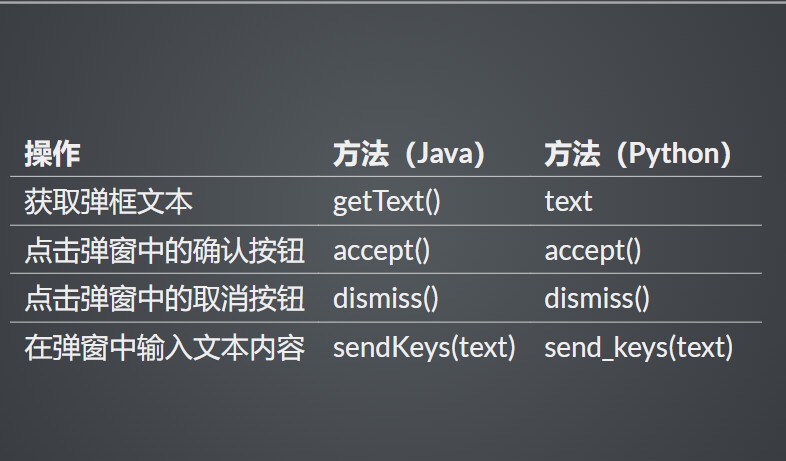
3,代码示例
//弹窗无法用元素定位
@Test
void alterHandle() throws InterruptedException {
driver.get("https://vip.ceshiren.com/#/ui_study/file_down");
//定位alter弹窗件按钮并点击弹出alter弹窗
WebElement warningBtn = driver.findElement(By.id("warning_btn"));
warningBtn.click();
sleep(3000);
//切换到alter弹窗页面
Alert alert = driver.switchTo().alert();
//获取alter页面文本
String alertText = alert.getText();
System.out.println("alert弹窗文本信息:" + alertText);
//点击alert弹窗的确认按钮
alert.accept();
// alert.dismiss(); //点击alert弹窗的取消按钮
assertThat(alertText,equalTo("alert哦,这是一条alert消息"));
}
//弹窗可以用元素定位
@Test
void alertElement() throws InterruptedException {
driver.get("https://vip.ceshiren.com/#/ui_study/file_down");
//定位元素按钮并点击两次弹出alter弹窗
WebElement primaryBtn = driver.findElement(By.id("primary_btn"));
primaryBtn.click();
primaryBtn.click();
sleep(3000);
String pageSource = driver.getPageSource();
//点击确定,接触弹窗操作
WebElement element = driver.findElement(By.xpath("//*[contains(text(),'确定')]"));
element.click();
sleep(3000);
assertTrue(pageSource.contains("该弹框点击两次后才会弹出"));
}