目录
- capability概述
- capability配置
- Selenium Grid简介
- 分布式运行
capability概述
capability 使用示例-Python
"""
=====Python版本=====
__author__ = '霍格沃兹测试开发学社'
__desc__ = '更多测试开发技术探讨,请访问:https://ceshiren.com/t/topic/15860'
"""
import time
from selenium import webdriver
from selenium.webdriver.common.by import By
def test_ceshiren():
# 切换成 windows 就会报错
capabilities = {"browserName":"chrome","platformName":"mac"}
# 通过 desired_capabilities 添加配置信息
driver = webdriver.Chrome(desired_capabilities=capabilities)
driver.implicitly_wait(5)
driver.get("https://ceshiren.com/")
# 输入框输入搜索内容[霍格沃兹测试学院]
text = driver.find_element(By.CSS_SELECTOR, ".login-button").text
# 点击搜索按钮
print(text)
time.sleep(30)
driver.quit()
capability 使用示例-Java
import org.junit.jupiter.api.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
/**
* @Author: 霍格沃兹测试开发学社
* @Desc: '更多测试开发技术探讨,请访问:https://ceshiren.com/t/topic/15860'
*/
public class CapabilityTest {
@Test
void ceshiren(){
ChromeOptions chromeOptions = new ChromeOptions();
chromeOptions.setCapability("platformName", "mac");
chromeOptions.setCapability("browserName", "chrome");
WebDriver driver = new ChromeDriver(chromeOptions);
driver.get("https://ceshiren.com/");
String text = driver.findElement(By.cssSelector(".login-button")).getText();
System.out.println(text);
driver.quit();
}
}
Selenium Grid
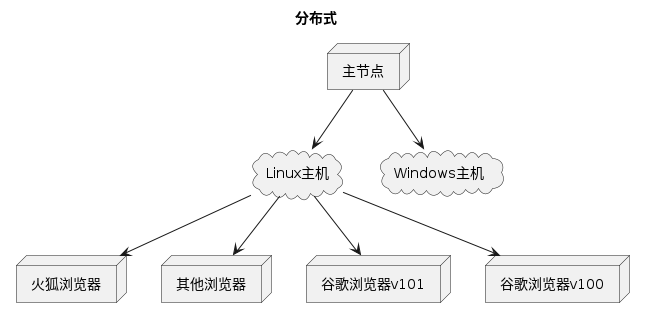
- Selenium Grid 允许我们在多台机器上并行运行测试,并集中管理不同的浏览器版本和浏览器配置(而不是在每个单独的测试中)。
- 官网地址:Grid | Selenium
演示环境
- 保证本地可以正常调通
- 实例化Remote()类并添加相应的配置
代码示例-Python
def test_ceshiren2():
hogwarts_grid_url = "https://selenium-node.hogwarts.ceshiren.com/wd/hub"
capabilities = {"browserName":"chrome","browserVersion":"101.0"}
# 配置信息
# 实例化Remote,获取可以远程控制的driver实例对象
# 通过 command_executor 配置selenium hub地址
# 通过 desired_capabilities 添加配置信息
driver = webdriver.Remote(
command_executor=hogwarts_grid_url,
desired_capabilities=capabilities)
driver.implicitly_wait(5)
driver.get("https://ceshiren.com/")
# 输入框输入搜索内容[霍格沃兹测试学院]
text = driver.find_element(By.CSS_SELECTOR, ".login-button").text
# 点击搜索按钮
print(text)
time.sleep(3)
driver.quit()
代码示例-Java
import org.junit.jupiter.api.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.MalformedURLException;
import java.net.URL;
/**
* @Author: 霍格沃兹测试开发学社
* @Desc: '更多测试开发技术探讨,请访问:https://ceshiren.com/t/topic/15860'
*/
public class CapabilityTest {
@Test
void remoteCeshiren() throws MalformedURLException {
ChromeOptions chromeOptions = new ChromeOptions();
chromeOptions.setCapability("browserVersion", "101.0");
chromeOptions.setCapability("browserName", "chrome");
WebDriver driver = new RemoteWebDriver(
new URL("https://selenium-node.hogwarts.ceshiren.com/wd/hub"),
chromeOptions);
driver.get("https://ceshiren.com/");
String text = driver.findElement(By.cssSelector(".login-button")).getText();
System.out.println(text);
driver.quit();
}
}