20230422_python编程语言
Python知识回顾.xmind (219.9 KB)
内置函数
https://docs.python.org/zh-cn/3/library/functions.html
- 封装:
提供访问
@property --把一个方法变成一个属性,直接使用.,不用加括号
提供修改
- 练习:
请设计一个自助咖啡机的人机交互系统,通过投币购买咖啡,需要实现以下功能:
咖啡机展示两种可选咖啡:拿铁咖啡和美式咖啡。格式如“拿铁(20元): 浓缩咖啡,牛奶,糖”
能够选择任意一种咖啡,并投入足够的钱币(金额不够时需要重新投币)。
咖啡机制作咖啡,并且记录本次交易记录(格式如“2023-03-24 16:24:49 订单:美式咖啡️,支付20元,找零5元”)。
咖啡机询问用户是否继续购买咖啡。如果继续,则从展示咖啡开始新流程。
如果用户不继续购买,咖啡机打印所有交易记录。
*设计思路:
- 需求分析:对象抽象成类,类有哪些属性和方法,设计类,提炼类的框架,方法和属性
方法三件套:名称、入参、返回值
静态分析,分析用到的类,有哪些属性和方法,定义好对应的名字,
动态思考,串起来之后,有哪些逻辑,谁先谁后,完整的交互流程
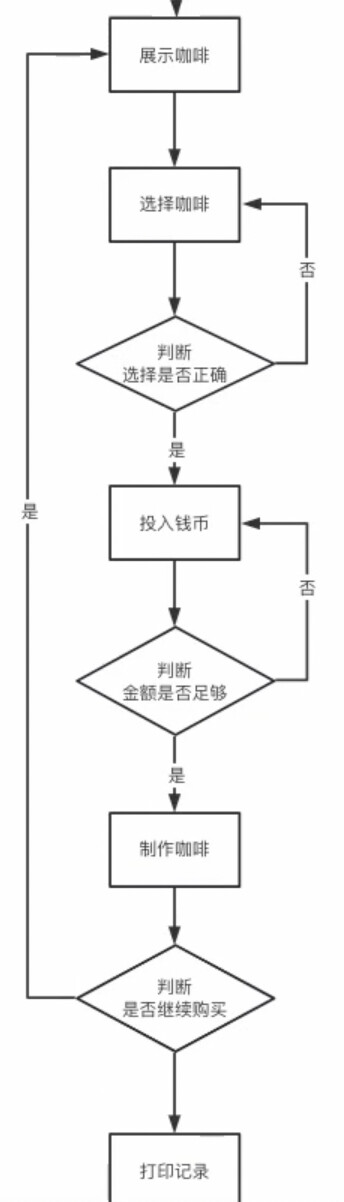
import time
from datetime import datetime
class Coffee:
def __init__(self, name: str, price: int, ingredient: str):
self.name = name
self.price = price
self.ingredient = ingredient
def __str__(self):
return f'{self.name}({self.price}元): {self.ingredient}'
class Latte(Coffee):
def __init__(self):
super().__init__('拿铁', 20, '浓缩咖啡,牛奶,糖')
class Americano(Coffee):
def __init__(self):
super().__init__('美式', 15, '浓缩咖啡')
class CoffeeMachine:
def __init__(self):
# 组合模式
self.products = [Latte(), Americano()]
self.records = []
def display(self):
"""展示咖啡"""
print('------ 展示咖啡 ------')
for coffee in self.products:
print(coffee)
def select_coffee(self) -> Coffee:
"""选择咖啡"""
print('------ 选择咖啡 ------')
while True:
selected = input('请输入数字选择咖啡 0:拿铁,1:美式咖啡')
if selected in ('0', '1'):
return self.products[int(selected)]
else:
print('输入不合法,请重新选择可选的咖啡。')
continue
def input_money(self, coffee: Coffee) -> int:
"""输入钱币"""
print('------ 输入钱币 ------')
while True:
money = input(f'请投入{coffee.price}元')
if int(money) < coffee.price:
print('金额不够,请重新投币。原金额已经退回')
continue
else:
return int(money)
def make_coffee(self, coffee: Coffee, money: int):
"""制作咖啡"""
print('------ 制作咖啡 ------')
print('咖啡制作中,请稍候...')
time.sleep(3)
now = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
record = f'{now} 订单:{coffee.name}咖啡️,支付{money}元,找零{money - coffee.price}元'
self.records.append(record)
def print_records(self):
"""打印记录"""
print('------ 打印记录 ------')
for rec in self.records:
print(rec)
if __name__ == '__main__':
machine = CoffeeMachine()
while True:
# 展示咖啡
machine.display()
# 选择咖啡
selected_coffee = machine.select_coffee()
# 投入钱币
inp_money = machine.input_money(selected_coffee)
# 制作咖啡
machine.make_coffee(selected_coffee, inp_money)
# 询问是否继续
answer = input('是否继续购买?Y:继续,N:不继续')
if answer == 'Y':
continue
else:
break
# 打印记录
machine.print_records()